Module 4: Add CSS to your adventure game
Objective: learn and use basic features of Cascading Style Sheets.
- CSS: adding style to your page
- CSS selectors
- Your very first stylesheet
- Comments: your #1 pal
- CSS classes and IDs
- Using Google Fonts
- Centering with Flexbox
- Fin!
CSS: adding style to your page
I hope all of your games are coming along smoothly! I'm sure you're eager to spice up your designs to look cooler than plain HTML, though. CSS (Cascading Style Sheets) is the tool to take care of that.

CSS is easy to pick up and can do very cool stuff off the bat. However, there is a great deal of depth to CSS as well. Due to the brevity of this course and its focus on game rather than visual design, we'll only touch the absolute basics of CSS. I highly recommend exploring it further on your own!
CSS selectors
CSS works by selecting elements from an HTML document
and adding properties to these elements. What are "elements?" Well, whenever you create a
set of tags, like <p>this paragraph</p>
, the browser creates an
element behind the scenes which can be manipulated by CSS (and, later, JavaScript).
The properties CSS allows you to modify range from size to color to positioning and to animation. I can only discuss a few basic properties; check w3schools' CSS reference for a list of properties you can add to HTML elements and go crazy experimenting.
Your very first stylesheet
CSS selectors can be placed in one of three ways: inside <style>
tags in
the <head>
of an HTML document, as an in-line attribute
right inside an HTML tag, and in a separate .css
file. You're welcome to do whichever you
want, but the most powerful method is the external file because you only need to make one
.css
file for your whole project, so that's what I'll focus on here.
Here's how to make your first stylesheet:
-
Create a new file in the same folder as your
index.html
file calledstyle.css
. -
Inside of
style.css
, write your first CSS selector:p { color: red; }
-
Save
style.css
, and head back to editing yourindex.html
file. Add the following element inside of the<head>
tags. This loads the CSS file and applies its selectors to the HTML document. You can reference the same stylesheet from as many HTML files as you want:<link rel="stylesheet" href="style.css">
And you're done! Open your index.html
file in a browser and you should see
all of your paragraphs have a red font color.
In your style.css
file, try changing p
to a
.
Refresh the website and see how the results change. Feel free to add as many selectors
as you like, and experiment with different properties.
Comments: your #1 pal
I also need to introduce comments, one of the most important parts of coding. Comments are mini explanations inside your code which maintain readability for yourself and your teammates. Here's a little example stylesheet, with comments, to whet your appetite for experimenting with different CSS properties:
/* The asterisk selector means "select all elements" */
* {
font-family: "Helvetica", sans-serif;
}
/* The body selector is a good place to add wallpaper */
body {
background: url("https://cdn.tutsplus.com/psd/uploads/legacy/001_WebDesignWeek/02_Layout/pattern_14.jpg");
}
a {
color: #41a6d8;
text-decoration: none;
}
/* :hover is a "pseudoselector" that becomes active
* whenever the mouse is in the element */
a:hover {
text-decoration: underline;
}
/* border is a somewhat more complex property, taking a
* size, a style, and a color separated by spaces */
p {
border: 1px solid #000000;
}
Note that colors are in hexadecimal format. I recommend using a color picker tool to generate these hexadecimal values and paste them into your stylesheet.
CSS classes and IDs
OK, so you now know how to apply CSS styles to all elements of a tag with a selector. But let's say you want to apply style to only a specific paragraph rather than all of your paragraphs at once, or want to create a single selector that you can apply to, say, paragraph, header, and anchor elements all at once. To do this, CSS provides classes and IDs, two powerful tools that work in similar ways. The only difference between the two is that you never have more than one element set to a given ID, but have as many elements sharing a class as you want.
In your CSS file, the syntax for making a class or ID is easy: simply make up any word you want and
prefix it with a #
to denote an ID and a .
to denote a class.
/* A class that centers text */
.center-text {
text-align: center;
}
/* An id for styling a particular huge button */
#huge-button {
font-size: 40px;
}
With IDs and classes under your belt, most deployment of CSS in HTML boils down to two
tags, <div>
and <span>
, which do nothing on their
own but act as generic elements to add CSS classes and IDs to. There is only one difference
between these tags: <div>
creates a line break and <span>
doesn't. Let's deploy the selectors we just made in a sample HTML file. I'll add CSS to the
header for brevity's sake. Note the class=""
and id=""
attributes:
<html>
<head>
<style>
.center-text {
text-align: center;
}
#huge-button {
font-size: 40px;
}
</style>
</head>
<body>
<div class="center-text">
<button id="huge-button">Click me!</button>
</div>
</body>
</html>
Last bit on IDs and classes: you can add as many classes to an element as you wish (separate each class name with a space), and you can combine an ID and classes on the same element.
Using Google Fonts
One of the coolest tools for web design is Google Fonts, which gives you instant access to a huge library of fonts without fuss. Using Google Fonts is a piece of cake.
-
Head over to Google Fonts and pick out a font you like.
-
Press the pink "+" icon to select it.
-
Expand the "1 Family Selected" toolbar that shows up at the bottom of the screen once you select a font.
-
Click "@IMPORT"
-
Copy and paste the line between the
<style>
tags and paste it at the top of yourstyle.css
file, outside of any selectors:@import url('https://fonts.googleapis.com/css?family=Bitter');
-
Copy and paste the
font-family
line into any selector(s) you wish:body { font-family: 'Bitter', serif; }
That's it! You can import as many fonts as you want. Click the "customize" tab to add different font styles and weights to your import if you choose. Here's a full example.
Centering with Flexbox
Until a tool called flexbox was added to CSS a couple years ago, centering items was pretty difficult. Flexbox is a fairly technical layout tool, so I'll only show you how to use it for centering purposes, for example, if you'd like to put content smack in the middle of the screen:
See the Pen flex centering by ggorlen (@ggorlen) on CodePen .
I won't bog you down with too much of an explanation here--just apply the .main
class to a <div>
and any elements inside of it will be centered
on both axes. The code inside of the body
selector ensures that
that container expands to fill the screen. vh
and vw
are CSS units
representing percentage of the viewport height and width.
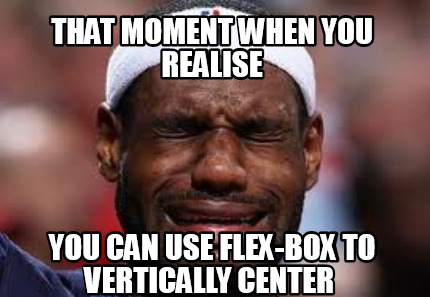
Fin!
OK, that should give you *plenty* of control over your HTML adventure game's design. Go at it!
Next module, we'll leave HTML and CSS behind and embark on JavaScript, which is really the meat of the class. If you have time, get a head start on JS over at Codecademy!
